XML Tutorial
Volume 9 : XSLT Functions
Tomoya Suzuki
Index
XSLT Control Commands
XML Documents Creation
Cautions when Writing XSLT Stylesheets
Review Questions
XSLT Control Commands
Programming-type selection processes, recursion processes, and other commands have been provided for use with XSLT stylesheets. Using these functions, a programmer can transform content according to certain conditions, or conduct repetitive processing.
Selection Processing
Selection processing can either take the form of "xsl:if command" (process is executed if conditions are "true") or "xsl:choose command" (process is executed for a first "true" condition at a conditional branch)
Conditional Process xsl:if Command
Using the xsl:if command, the template written within the xsl:if command is executed if the results of an XPath method described in a test attribute are true.
If the condition is not true, then the template is not executed. There is no template description in XSLT that corresponds to the programming language "~else~" if the conditions are not true. In this case, use the xsl:choose command, introduced below.
Let’s take a look at an example of an xsl:if command. We will apply an XSLT stylesheet (LIST2) to a source XML document (LIST1):
LIST1: Source XML Document (list1.xml)
01 <?xml version="1.0"?>
02 <?xml-stylesheet type="text/xsl" href="list2.xsl"?>
03 <ProductList>
04 <Product Code="XML001" NewProduct="true">
05 <ProductName>XML Ballpoint Pen</ProductName>
06 <UnitPrice>500</UnitPrice>
07 </Product>
08 <Product Code="XML002">
09 <ProductName>XML Mousepad</ProductName>
10 <UnitPrice>150</UnitPrice>
11 </Product>
12 </ProductList>
LIST2:XSLT Stylesheet(list2.xsl)
01 <?xml version="1.0"?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <html>
06 <body>
07 <h1>ProductList</h1>
08 <table border="1">
09 <tr><th>Product Name</th><th>UnitPrice</th></tr>
10 <xsl:apply-templates select="ProductList/Product" />
11 </table>
12 </body>
13 </html>
14 </xsl:template>
15 <xsl:template match="Product">
16 <tr>
17 <td>
18 <xsl:if test="@NewProduct='true'" >[NewProduct]</xsl:if>
19 <xsl:value-of select="ProductName" />
20 </td>
21 <td><xsl:value-of select="UnitPrice" /></td>
22 </tr>
23 </xsl:template>
24 </xsl:stylesheet>
The example above is a stylesheet for creating HTML to display products in a list. Here, when the attribute value of the NewProduct attribute is "true," a "NewProduct" mark is displayed on the list (LIST2, line 18). When we want to display something only when certain conditions are met, we select the xsl:if command to provide the functionality.
Conditional Branch Processing xsl:choose Command
Use the xsl:choose command when you want to select from a collection of templates based on certain conditions.
The xsl:when command is used as the child element of the xsl:choose command. If the test attribute designated in the xsl:when command is "true," the template inside the xsl:when command is executed. Several xsl:when commands can be incorporated at the same time; the template associated with the first "true" xsl:when command will be executed.
A single xsl:otherwise command may also be used within the xsl:choose command. If there are no "true" conditions for an xsl:when test attribute, the template inside the xsl:otherwise command is executed. Nothing is executed if there is no "true" condition for an xsl:when test attribute, and no xsl:otherwise command has been included.
The LIST3 XML document contains a Remarks element (line 7, line 12); the LIST4 XSLT stylesheet is applied to this document, outputting its content in HTML format.
LIST3:XML Document(list3.xml)
01 <?xml version="1.0" ?>
02 <?xml-stylesheet type="text/xsl" href="list4.xsl"?>
03 <ProductList>
04 <Product Code="XML001">
05 <ProductName>XML Ballpoint Pen</ProductName>
06 <UnitPrice>500</UnitPrice>
07 <Remarks>Limited to 30 people</Remarks>
08 </Product>
09 <Product Code="XML002">
10 <ProductName>XML Mousepad</ProductName>
11 <UnitPrice>150</UnitPrice>
12 <Remarks/>
13 </Product>
14 </ProductList>
LIST4:XSLT Stylesheet(list4.xsl)
01 <?xml version="1.0" ?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <html>
06 <body>
07 <h1>ProductList</h1>
08 <table border="1">
09 <tr><th>Product Name</th><th>UnitPrice</th><th>Remarks⇒
</th></tr>
10 <xsl:apply-templates select="ProductList/Product" />
11 </table>
12 </body>
13 </html>
14 </xsl:template>
15 <xsl:template match="Product">
16 <tr>
17 <td><xsl:value-of select="ProductName" /></td>
18 <td><xsl:value-of select="UnitPrice" /></td>
19 <xsl:choose>
20 <xsl:when test="Remarks/text()">
21 <td><xsl:value-of select="Remarks" /></td>
22 </xsl:when>
23 <xsl:otherwise>
24 <td>No remarks</td>
25 </xsl:otherwise>
26 </xsl:choose>
27 </tr>
28 </xsl:template>
29 </xsl:stylesheet>
Here, any text contained in the Remarks element will be output; otherwise, the message "No remarks" will be output.
Assume that the LIST6 XSLT stylesheet is applied to LIST5, outputting sales information in HTML format:
LIST5:XML Document(list5.xml)
01 <?xml version="1.0" ?>
02 <?xml-stylesheet type="text/xsl" href="list6.xsl"?>
03 <SalesChart>
04 <Product Code="XML001">
05 <ProductName>XML Ballpoint Pen</ProductName>
06 <UnitPrice>500</UnitPrice>
07 <Volume>2</Volume>
08 </Product>
09 <Product Code="XML002">
10 <ProductName>XML Mousepad</ProductName>
11 <UnitPrice>150</UnitPrice>
12 <Volume>18</Volume>
13 </Product>
14 <Product Code="XML003">
15 <ProductName>XML Strap</ProductName>
16 <UnitPrice>150</UnitPrice>
17 <Volume>13</Volume>
18 </Product>
19 </SalesChart>
LIST6:XSLT Stylesheet(list6.xsl)
01 <?xml version="1.0" ?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <html>
06 <body>
07 <h1>Sales List</h1>
08 <table border="1">
09 <tr><th>Product Name</th><th>UnitPrice</th><th>Volume</th></tr>
10 <xsl:apply-templates select="SalesChart/Product" />
11 </table>
12 </body>
13 </html>
14 </xsl:template>
15 <xsl:template match="Product">
16 <tr>
17 <td><xsl:value-of select="ProductName" /></td>
18 <td><xsl:value-of select="UnitPrice" /></td>
19 <xsl:choose>
20 <xsl:when test="Volume>15">
21 <td bgcolor="lightgreen"><xsl:value-of select= "Volume" /></td>
22 </xsl:when>
23 <xsl:when test="Volume>10">
24 <td bgcolor="lightblue"><xsl:value-of select= "Volume" /></td>
25 </xsl:when>
26 <xsl:otherwise>
27 <td><xsl:value-of select="Volume" /></td>
28 </xsl:otherwise>
29 </xsl:choose>
30 </tr>
31 </xsl:template>
32 </xsl:stylesheet>
Here, the bgcolor attribute changes the displayed background color according to sales volume. Under normal conditions, no background color is displayed.
Recursive Processing
Use the "xsl:apply-templates" command or "xsl:for-each" command to process templates repetitively for each selected node.
Repetitive Processing xsl:for-each Command
Define an XPath forming a node set in the select attribute of the xsl:for-each command, and repeat the execution of templates within the xsl:for-each for each selected node.
For example, let’s apply the XSLT stylesheet in LIST8 to the seminar information described in LIST7, outputting the results in HTML:
LIST7:XML Document(list7.xml)
01 <?xml version="1.0" ?>
02 <?xml-stylesheet type="text/xsl" href="list8.xsl"?>
03 <SeminarDetails>
04 <CourseName>XML Introduction</CourseName>
05 <Code>1234</Code>
06 <Content>
07 <Chapter>XML Overview</Chapter>
08 <Chapter>XML Document Creation</Chapter>
09 <Chapter>DTD</Chapter>
10 <Chapter>XML Schema</Chapter>
11 <Chapter>XSLT, XPath</Chapter>
12 <Chapter>Namespace</Chapter>
13 </Content>
14 </SeminarDetails>
LIST8:XSLT Stylesheet(list8.xsl)
01 <?xml version="1.0" ?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <html>
06 <body>
07 <h1><xsl:value-of select="SeminarDetails/CourseName" /></h1>
08 CourseName:<xsl:value-of select="SeminarDetails/CourseName" /><br/>
09 CourseCode:<xsl:value-of select="SeminarDetails/Code" /><br/>
10 Content:<br/>
11 <xsl:for-each select="SeminarDetails/Content/Chapter">
12 - <xsl:value-of select="." /><br/>
13 </xsl:for-each>
14 </body>
15 </html>
16 </xsl:template>
17 </xsl:stylesheet>
Here, a repeated list is output for each Chapter element within the Content element. The node selected in the xsl:for-each command serves as the current node; we must be careful of this when designating the internal relative path (LIST8, line 12).
Difference between xsl:apply-templates and xsl:for-each
With the xsl:apply-templates command, xsl:template elements are individually applied to the selected node set. This provides the same type of recursive processing as the xsl:for-each command. The xsl:for-each command is suited to expressing small-scale repetitive processes, and is very convenient when outputting simple sequences of small data categories located at the end from within a collection of data.
However, when repeating processes on major data categories, the template rule used when transforming smaller data categories becomes rather unwieldy, and attempting to use the xsl:for-each command to accomplish repetitive processing results in a lengthy notation for the command itself. This method also involves using a complex nested structure, resulting in many layers of indents. Attempting to use only the xsl:for-each command for all repetitive processes creates a stylesheet that is difficult to follow intuitively.
Instead, we can create a single template rule with the xsl:template element for the data category we wish to display. This method is similar to a programming subroutine. We apply this method to the categories selected using the xsl:apply-templates command. Separating each category to be displayed by template rules makes the entire sequence much easier to understand. When using the xsl:for-each command, a single template (included within the command) is repeated. On the other hand, an XPath utilizing a predicate can be designated in the match attribute of the xsl:template element, allowing the programmer to switch among template rules.
The xsl:choose command can be combined inside the xsl:for-each command to allow for branching; however, the notation can become quite long and involved. Programmers should use their discretion here, selecting the best method according to the length of the notation required.
XML Document Creation
XSLT can be used to transform a source XML document into a document having a different structure. In prior volumes, we mainly discussed transforming documents into HTML format, but transformations can be made into other formats, including XML documents, text documents, or XML documents of differing formats. Portions of documents can be extracted and used for other purposes, output into CSV format, etc.
Creating Elements and Attributes
Writing elements and attributes directly within a template rule allows for the output of elements and attributes as transformation results.
LIST9:XML Document(list9.xml)
1 <?xml version="1.0" ?>
2 <?xml-stylesheet type="text/xsl" href="list10.xsl"?>
3 <ProductList>
4 <Product Code ="XML001">
5 <ProductName>XML Ballpoint Pen</ProductName>
6 <UnitPrice >500</UnitPrice>
7 </Product>
8 </ProductList>
LIST10:XSLT Stylesheet(list10.xsl)
01 <?xml version="1.0" ?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <products>
06 <item>
07 <name><xsl:value-of select="ProductList/Product/ProductName" /></name>
08 <price><xsl:value-of select="ProductList/Product/UnitPrice" /></price>
09 </item>
10 </products>
11 </xsl:template>
12 </xsl:stylesheet>
Further, the xsl:element command and xsl:attribute command can be used to output elements and attributes that are assigned arbitrary names. With the xsl:element command and xsl:attribute command, the value designated in the name attribute can be output as element or attribute names.
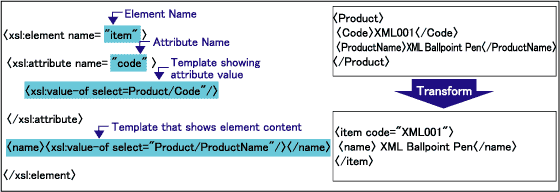
Use the xsl:element command or xsl:attribute command to determine an element name based on information in a source XML document. It is also possible to transform an element into an attribute or vice-versa.
Transforming an Attribute into an Element
Data described as an attribute in a source XML document can be output as an element. In the XSLT stylesheet shown in LIST10, the element is directly described. In the XSLT stylesheet shown in LIST11, the xsl:element command is used to output the Code attribute (LIST9, line 4) in the source XML document as an element (LIST11, lines 7 through 9).
LIST11:XSLT Stylesheet(list11.xsl)
01 <?xml version="1.0"?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <xsl:element name="ProductList">
06 <xsl:element name="Product">
07 <xsl:element name="Code">
08 <xsl:value-of select="ProductList/Product/@Code" />
09 </xsl:element>
10 <xsl:element name="ProductName">
11 <xsl:value-of select="ProductList/Product/ProductName" />
12 </xsl:element>
13 <xsl:element name="UnitPrice">
14 <xsl:value-of select="ProductList/Product/UnitPrice" />
15 </xsl:element>
16 </xsl:element>
17 </xsl:element>
18 </xsl:template>
19 </xsl:stylesheet>
Copying Nodes
When transforming one XML document into a separate XML document, there are times when the original XML document can be used as is. In these cases, use the "xsl:copy-of" command or "xsl:copy" command to copy elements and/ or attributes from the original XML document.
xsl:copy-of Command
Copy the entire node and child node designated in the select attribute of the xsl:copy-of command.
For example, let’s assume that we apply an XSLT stylesheet (LIST13) that will only extract the Product element having a Code of "XML001" from the XML document in which the ProductName is described (LIST12).
LIST12:XML Document (list12.xml)
01 <?xml version="1.0"?>
02 <?xml-stylesheet type="text/xsl" href="list13.xsl"?>
03 <SalesChart>
04 <Product Code="XML001">
05 <ProductName>XML Ballpoint Pen</ProductName>
06 <UnitPrice>500</UnitPrice>
07 <Volume>2</Volume>
08 </Product>
09 <Product Code="XML002">
10 <ProductName>XML Mousepad</ProductName>
11 <UnitPrice>150</UnitPrice>
12 <Volume>18</Volume>
13 </Product>
14 <Product Code="XML003">
15 <ProductName>XML Strap</ProductName>
16 <UnitPrice>150</UnitPrice>
17 <Volume>13</Volume>
18 </Product>
19 </SalesChart>
LIST13:XSLT Stylesheet(list13.xsl)
1 <?xml version="1.0"?>
2 <xsl:stylesheet version="1.0"
3 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
4 <xsl:template match="/">
5 <ProductInformation>
6 <xsl:copy-of select="SalesChart/Product[@Code='XML001']"/>
7 </ProductInformation>
8 </xsl:template>
9 </xsl:stylesheet>
In this case, the xsl:copy-of command is used to make a bulk copy of the applicable Product element, and output the results (LIST14).
LIST14: Transformation Results(list14.xml)
01 <?xml version="1.0" ?>
02 <ProductInformation>
03 <Product Code="XML001">
04 <ProductName>XML Ballpoint Pen</ProductName>
05 <UnitPrice>500</UnitPrice>
06 <Volume>2</Volume>
07 </Product>
08 </ProductInformation>
xsl:copy Command
While the xsl:copy-of command is used for bulk copying, the xsl:copy command is used to copy discrete sections of data, add separate elements, or copy in very small, detailed units. This command copies only the current node.
Copying can be repeated recursively when the xsl:copy command is used in combination with template rules.
For example, lines 9 through 13 in LIST15 show nodes being copied recursively.
LIST15:XSLT Stylesheet(list15.xsl)
01 <?xml version="1.0" ?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <ProductInformation>
06 <xsl:apply-templates select="SalesChart/Product[@Code='XML001']"/>
07 </ProductInformation>
08 </xsl:template>
09 <xsl:template match="*|text()">
10 <xsl:copy>
11 <xsl:apply-templates select="node()|@*"/>
12 </xsl:copy>
13 </xsl:template>
14 <xsl:template match="Volume">
15 </xsl:template>
16 <xsl:template match="@Code">
17 <xsl:element name="{name(.)}">
18 <xsl:value-of select="."/>
19 </xsl:element>
20 </xsl:template>
21 </xsl:stylesheet>
In lines 16 through 20, a template rule is applied to the Code attribute, and the attribute is transformed into an element and copied. The template rule in lines 14 and 15 is applied to the Volume element; however, the template rule is empty, so nothing is copied (LIST16).
LIST16:Transformation Results(list16.xml)
01 <?xml version="1.0" ?>
02 <ProductInformation>
03 <Product>
04 <Code> XML001 </Code>
05 <ProductName>XML Ballpoint Pen</ProductName>
06 <UnitPrice>500</UnitPrice>
07 </Product>
08 </ProductInformation>
Storing Values using the xsl:param Element
The xsl:param element can be used to associate and declare a variable name and value. This allows a conditional value to be represented by a variable name rather than a complex XPath, providing for notation clarity. Either of the following two methods can be used to associate a variable name and a value:
(1) Designate a value using the select attribute
Designate the value in the select attribute of the xsl:param element (LIST17, line 18). When using XPath to make the designation, the attribute value template is used.
LIST17:XSLT Stylesheet(list17.xsl)
01 <?xml version="1.0" ?>
02 <xsl:stylesheet version="1.0"
03 xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
04 <xsl:template match="/">
05 <html>
06 <body>
07 <h1>Sales List</h1>
08 <table border="1">
09 <tr><th>Product Name</th><th>UnitPrice</th><th>Volume</th></tr>
10 <xsl:apply-templates select="SalesChart/Product" >
11 <xsl:with-param name="key" select="10"/>
12 </xsl:apply-templates>
13 </table>
14 </body>
15 </html>
16 </xsl:template>
17 <xsl:template match="Product">
18 <xsl:param name="key" select="20"/>
19 <xsl:if test="Volume>$key">
20 <tr>
21 <td><xsl:value-of select="ProductName" /></td>
22 <td><xsl:value-of select="UnitPrice" /></td>
23 <td><xsl:value-of select="Volume" /></td>
24 </tr>
25 </xsl:if>
26 </xsl:template>
27 </xsl:stylesheet>
(2) Designate a value using a template
Designate a value as a child element of the xsl:param element. A tree structure element may also be designated as a template.
Using Variables
Variables may be designated as "$VariableName" (LIST17, line 19). Use the xsl:value-of command to output a value, or the predicate to designate a conditional value. A variable associated with a tree structure can be designated in an xsl:copy-of command, and output as transformation results.
xsl:with-param Value Passed using an Element
By describing the xsl:with-param element as the child element of the xsl:apply-templates command, we can pass over the value for the variable designated with xsl:param in the applied template rule (LIST17, line 11). The xsl:with-param element notation is the same as that for the xsl:param element:
If there are no related parameters in the template applied, it will be ignored.
Passing Values from an External Program
A value associated with the xsl:param element variable can be defined from a program that processes the XSLT stylesheet. In so doing, we can temporarily hold a conditional value as a variable, and then change it to transformed content by designating the value at the time of execution.
For example, we can create an XSLT stylesheet that extracts information related to a single Product from the ProductList according to ProductCode. Then, we can extract any Product data we wish by designating the ProductCode for extraction from outside the program. There are various development environment APIs available that can be used to designate targets from outside the program.
Cautions when Writing XSLT Stylesheets
Lastly, let’s talk about a few things to remember when actually writing an XSLT stylesheet on your own.
Create a Well-Formed Document
An XSLT stylesheet is an XML document. As such, at the very least it must be a well-formed document. The empty element "/" is particularly easy to forget. The xsl:value-of and xsl:apply-templates commands are normally empty elements. Also pay particular attention to the br and img HTML tags, since they must be notated as empty elements.
Correctly Define XPath
If you make a mistake regarding the current node, you will not be able to create a correct XPath method. Always be aware of what the current node is when you work, or the following types of errors can occur:
●Nothing is displayed
If an incorrect XPath method is used for the select attribute of the xsl:value-of command, nothing will be output. Nothing will be displayed, either, if an incorrect XPath method is used in the select attribute of the xsl:apply-templates command. This is because no node will be selected, and no template rule will be applied. Since the desired template rule is not processed, then nothing will be displayed for that portion.
●Text content of the source XML document is continuously displayed
Using an incorrect XPath method in the match attribute of the xsl:templates command means there will be no template rule to apply to the node selected. Accordingly, the built-in template rule for the selected node set will be applied, and the text content will be displayed continuously.
Review Questions
Question 1
Which of the following is incorrect with respect to XSLT functions?
- The xsl:if command includes a description of a template that is executed if the test attribute condition is "true"
- The xsl:for-each command allows repetitive processing for all nodes defined in the select attribute
- The xsl:choose command incorporates the processing of an xsl:else element when there are no "true" conditions
- The xsl:copy command only copies the current node
Comments
The xsl:choose command processes the first template for conditions in which the formula in the test attribute of the xsl:when element is "true". If there are no "true" conditions, the template for the xsl:otherwise element is processed. Accordingly, the incorrect description is C.
Question 2
Assume that the XSLT Stylesheet is applied to XML Document A, resulting in the output of XML Document B. Select the section of the XSLT stylesheet that correctly fits into (1) below.
[XML Document A]
<?xml version="1.0" ?>
<Product>
<Orange UnitPrice="40"/>
</Product>
[XSLT Stylesheet]
<?xml version="1.0" ?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<Fruit>
<xsl:apply-templates select="/Product/Orange"/>
</Fruit>
</xsl:template>
<xsl:template match="Orange">
<Orange>
</Orange>
</xsl:template>
</xsl:stylesheet>
[XML Document B]
<?xml version="1.0" ?>
<Fruit>
<Orange><UnitPrice>40</UnitPrice></Orange>
</Fruit>
A.
<xsl:attribute name="UnitPrice">
<xsl:value-of select="UnitPrice"/>
</xsl:attribute>
B.
<xsl:element name="UnitPrice">
<xsl:value-of select="UnitPrice"/>
</xsl:element>
C.
<xsl:attribute name="UnitPrice">
<xsl:value-of select="@UnitPrice"/>
</xsl:attribute>
D.
<xsl:element name="UnitPrice">
<xsl:value-of select="@UnitPrice"/>
</xsl:element>
Comments
In XML Document B (the output result), the UnitPrice element is described as the child element of the Orange element. Accordingly, the correct notation for (1) is the notation that creates the UnitPrice element. The xsl:element command is the function that creates elements. The xsl:attribute command is the function that creates attributes.
To output element content, the xsl:value-of command is used, with the XPath method showing the output value included in the select attribute. Since the UnitPrice attribute is written in the source XML document, the "@" symbol must be attached. Accordingly, the correct answer is D.
Question 3
Assume that the XSLT stylesheet is applied to XML Document A, resulting in the output of XML Document B. Select the section of the XSLT stylesheet that correctly fits into (1) below.
[XML Document A]
<?xml version="1.0" ?>
<item>
<itemName>XML Ballpoint Pen</itemName>
<itemNo>PN001</itemNo>
<size unit="cm">15</size>
</item>
[XSLT Stylesheet]
<?xml version="1.0" ?>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<xsl:apply-templates select="item"/>
</xsl:template>
<xsl:template match="item">
</xsl:template>
</xsl:stylesheet>
[XML Document B]
<?xml version="1.0" ?>
<size unit="cm">15</size>
- <xsl:copy-of select="size"/>
- <xsl:copy select="size"/>
- <xsl:copy-of match="size"/>
- <xsl:element name="size"/>
Comments
The xsl:copy-of command copies all of the descendant nodes that match the XPath method written in the select attribute (including attributes and element content). The xsl:copy command only copies the current node, and there is not select attribute definition. The xsl:element command is only used to create elements. Accordingly, the correct answer is A.
Tomoya Suzuki
Toshiba OA Consultant, Ltd. Training Solutions Engineering Department. Mr. Suzuki works mainly as an instructor in XML and programming language seminars. He laments that, for some reason, all of the rainy days during rainy season were on the weekends this year, preventing him from playing tennis, and causing him to suffer from a lack of exercise. He does predict, however, that he will have a deep, dark tan by the time this article is published. Don’t forget the sun block, Tomoya.
The content presented here is an HTML version of an article that originally appeared in the November 2007 issue of DB Magazine published by Shoeisya.